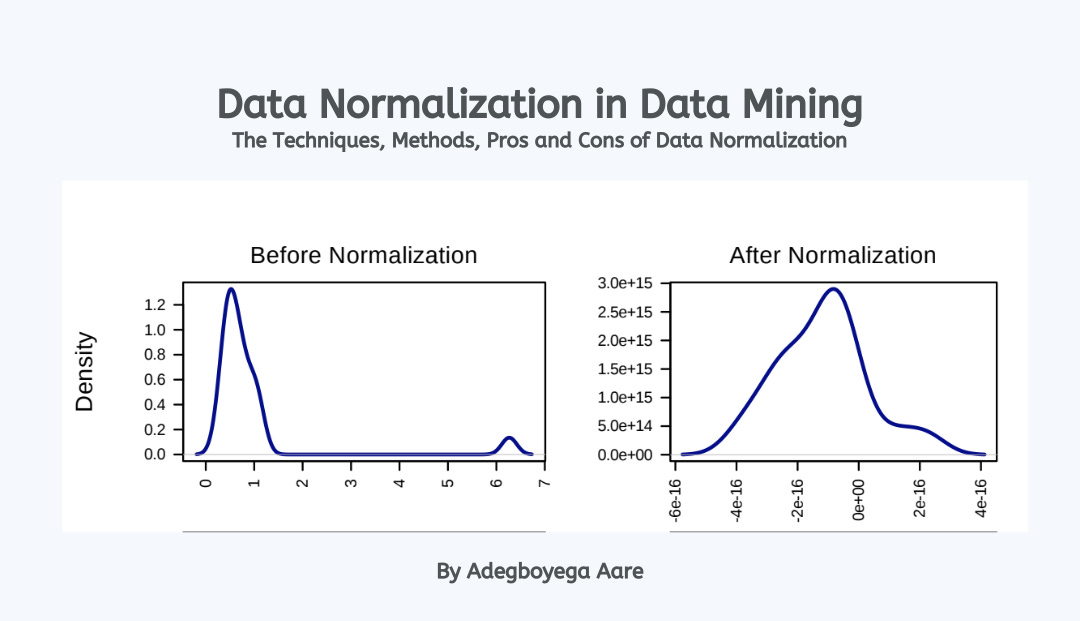
Normalization is an important step when working with data in data mining. Its main goal is to make the data easier to work with by putting it into a consistent format while still keeping its important details. This is necessary because data sets usually have numbers that are in different units, have different scales, and are spread out. These differences can make it hard to compare and analyze the data.
In this article, we'll look at various data normalization techniques, how to do it, the advantages and disadvantages, and answer common questions about normalization in data mining.
Techniques used in Data normalization
There are several techniques commonly used to normalize data:
1. Min-Max Scaling
Min-max scaling (also known as feature scaling) transforms data to a specified range, typically [0, 1]. The formula for Min-Max scaling is:
X_normalized = (X - X_min) / (X_max - X_min)
Where;
X: The original data point.
X_min: The minimum value in the dataset.
X_max: The maximum value in the dataset.
from sklearn.preprocessing import MinMax
Scalerscaler = MinMaxScaler()
normalized_data = scaler.fit_transform(data)
Min-max scaling is handy when you want to keep the relative differences between values.
2. Z-Score Standardization
Z-Score standardization (also known as standard score) scales data to have a mean of 0 and a standard deviation of 1. It is particularly useful when dealing with normally distributed data. The formula for Z-Score standardization is:
X_normalized = (X - X_mean) / X_stddev
Where;
X: The original data point.
μ (mu): The mean of the dataset.
σ (sigma): The standard deviation of the dataset.
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
normalized_data = scaler.fit_transform(data)
3. Log Transformation
Log transformation is applied to data with a highly skewed distribution. It helps make the data more symmetric and manageable. The formula is:
X_normalized = log(X)
import numpy as np
normalized_data = np.log(data)
4. Robust Scaling: This method is like Min-Max scaling but is less influenced by extreme values. It keeps data in a range while considering outliers.
5. Decimal Scaling: Decimal scaling moves the decimal point in values to a common scale. For example, all values might have only one decimal place.
You can pick the one that suits your data and goals. Each method has its pros and cons, so choose based on your specific data and what you want to achieve.
Why and When to Normalize Data?
Take a look at my earlier article titled "Introduction to Normalization & Standardization" for a thorough explanation of why we use data normalization in data mining and why it's important.
Advantages of Normalization
1. Improved Model Performance: Normalizing data makes model predictions more accurate and stable, especially when dealing with variables of different scales.
2. Faster Convergence: Normalization helps optimization algorithms work better by ensuring all variables contribute evenly to the learning process, speeding up convergence.
3. Robustness to Outliers: It can make models more resilient to extreme values, making the analysis more reliable.
Disadvantages of Normalization
1. Loss of Original Meaning: Normalization changes data, making it harder to understand the original values of variables.
2. Assumption of Linearity: Some normalization methods assume a straight-line relationship between variables, which may not be true for complex data.
3. Sensitivity to Scaling Range: Choosing the normalization range (like 0-1 or -1 to 1) can affect results and may require domain-specific knowledge.
FAQs on Data Normalization
Q1: Is Data Normalization Always Necessary?
Normalization is a good practice in data mining, but it's not always a must-do. Depending on your data and what you want to find, you might skip it sometimes.
Q2: Can I Normalize Different Parts of My Data Differently?
Yes, you can! It's okay to use different ways to normalize data if your data's different parts need it.
Q3: Should I Normalize Categorical Data?
Normally, you'd normalize numbers, not categories. For categorical data, you'd use different tricks, like one-hot encoding.
In summary, data normalization is important in data mining. It helps get your data ready for analysis. Knowing how to do it right can help you find valuable insights.
For those of you new to my newsletter - follow me on LinkedIn, hit me up on Twitter and follow my Facebook Page.
To support me as I roll out more content weekly, click HERE to buy me a coffee.
This post is public so feel free to share it, Thank you for reading…