Simplifying SQL Concepts: CTEs, Views, and Stored Procedures
Getting Started WIth SQL CTEs, Views, and Stored Procedures
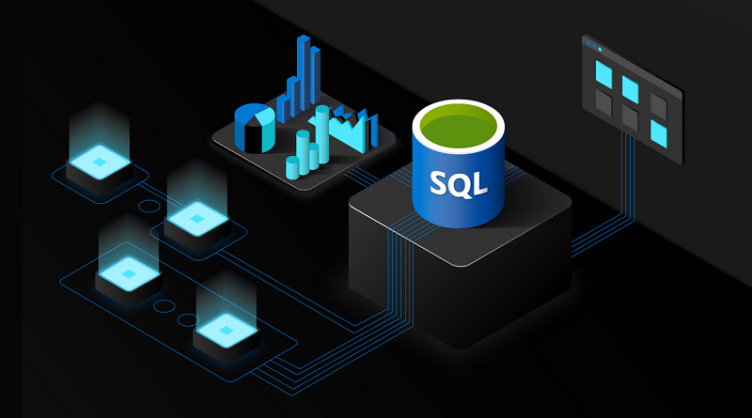
In the world of databases, SQL (Structured Query Language) offers a trio of powerful tools: Common Table Expressions (CTEs), Views, and Stored Procedures. These tools make database management easier, more efficient, and organized. Let's break down what they are and how they can benefit you.
Common Table Expressions (CTEs)
Common Table Expression (CTE) is like a helper table that you can use to make your complex queries simpler and more understandable. Imagine you're working with a list of employees and their salaries, and you want to find out who earns more than the average salary. A CTE can make this task easier.
Here's a basic example using a CTE and some sample data:
Sample Data:
Let's create a simple table called "Employees" with two columns, "Name" and "Salary."
CREATE TABLE Employees (
Name VARCHAR(50),
Salary INT
);
INSERT INTO Employees (Name, Salary)
VALUES
('Alice', 50000),
('Bob', 60000),
('Charlie', 55000),
('David', 70000),
('Eve', 48000);
Now, you can use a CTE to find employees who earn more than the average salary:
-- Using a CTE to find employees earning more than the average salary
WITH AverageSalary AS (
SELECT AVG(Salary) AS AvgSalary FROM Employees
)
SELECT Name, Salary
FROM Employees
WHERE Salary > (SELECT AvgSalary FROM AverageSalary);
In this example, the CTE "AverageSalary" calculates the average salary, and then we use it in the main query to find employees earning more than that average. It makes the query easier to understand and manage.
Stored Procedures
SQL-stored Procedure is like a reusable script that you can use to perform specific tasks. It's handy when you have a series of SQL statements that need to be executed together. Let's illustrate with an example:
Creating a Stored Procedure:
Suppose you want to create a stored procedure that adds a new employee to the "Employees" table. Here's how you can do it:
-- Creating a stored procedure to add a new employee
CREATE PROCEDURE AddNewEmployee (
@Name VARCHAR(50),
@Salary INT
)
AS
BEGIN
INSERT INTO Employees (Name, Salary)
VALUES (@Name, @Salary);
END;
Using the Stored Procedure:
Now, whenever you want to add a new employee, you can simply call the stored procedure:
-- Calling the stored procedure to add a new employee
EXEC AddNewEmployee @Name = 'Frank', @Salary = 65000;
This stored procedure streamlines the process of adding new employees to your database.
Views
SQL Views is like a saved query that you can reuse. It simplifies complex queries and saves you from writing the same code repeatedly. Let's consider an example using a view:
Sample Data:
Suppose you have a table called "Orders" with columns "OrderID," "CustomerName," and "OrderAmount."
CREATE TABLE Orders (
OrderID INT,
CustomerName VARCHAR(50),
OrderAmount DECIMAL(10, 2)
);
INSERT INTO Orders (OrderID, CustomerName, OrderAmount)
VALUES
(1, 'Alice', 100.50),
(2, 'Bob', 75.25),
(3, 'Charlie', 200.00),
(4, 'David', 50.75),
(5, 'Eve', 300.25);
Now, let's create a view to easily see the total order amount for each customer:
-- Creating a view to calculate total order amount for each customer
CREATE VIEW CustomerOrderTotal AS
SELECT CustomerName, SUM(OrderAmount) AS TotalAmount
FROM Orders
GROUP BY CustomerName;
With the view in place, you can simply query it whenever you need to see the total order amounts for each customer:
-- Using the view to see total order amounts for each customer
SELECT * FROM CustomerOrderTotal;
This view makes it much simpler to get this information without rewriting the complex query.
In summary, Common Table Expressions (CTEs), Views, and Stored Procedures are valuable tools in SQL that simplify complex tasks, make queries more readable, and streamline common database operations. They enhance the efficiency and maintainability of your database work.
Your support is invaluable
Did you like this article? Then please leave a share or even a comment, it would mean the world to me!
Don’t forget to subscribe to my YouTube account HERE, Where you will get a video explaining this article!