Top Key Statistical Concepts for Data Science
Learn Key Statistics in Data Science with Practical Python Examples
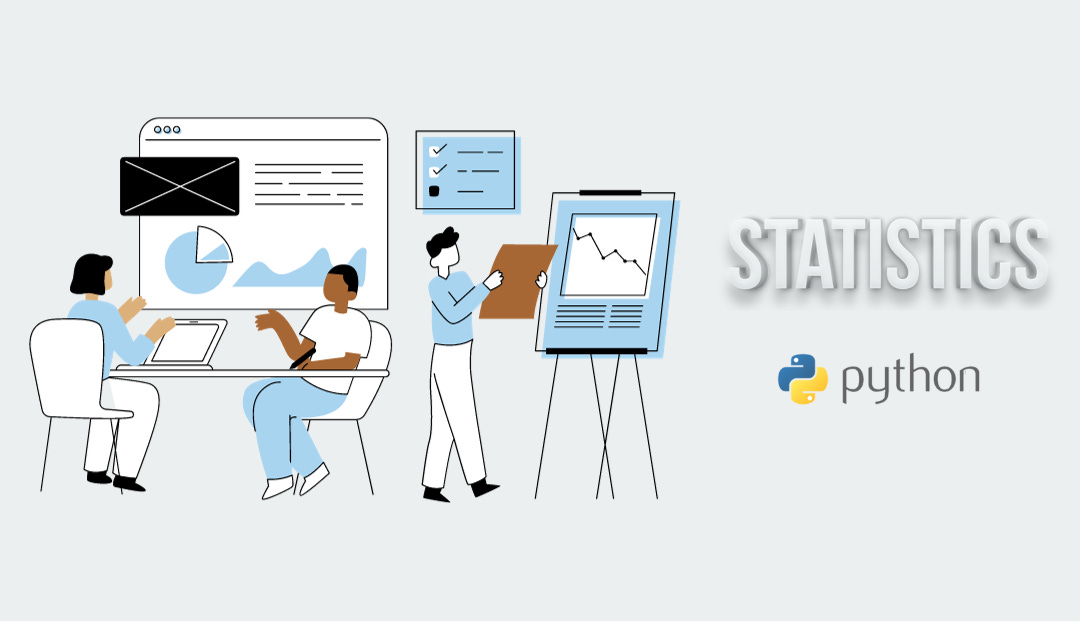
Statistics is like the starting point for data science. It's the tool that helps us understand data better. Whether you're trying to figure out what's happening in the market, make predictions about the future, or make sense of a small chunk of data, knowing about statistics is super important. In this article, we will delve into key statistical concepts and applications in data science. We'll also use Python to show practical examples to make it easier to understand.
Descriptive Statistics
Descriptive statistics are tools that help us understand data better. They include things like the average, middle value, most common value, and how spread out the data is. With Python libraries like NumPy and Pandas, you can find and show these statistics.
Python Code Syntax:
import numpy as np
data = np.array([12, 15, 18, 21, 24, 27, 30])
mean = np.mean(data)
median = np.median(data)
mode = np.mode(data)
Use Case: Calculate and display the central tendencies of a dataset to understand the distribution of student ages in a class.
Inferential Statistics
Inferential statistics involves drawing conclusions about a population from a sample. Methods like hypothesis testing and confidence intervals are used for Inferential statistics. Python's SciPy library has tools that help us do inferential statistics.
Python Code Syntax:
from scipy import stats
sample_data = [5, 6, 7, 8, 9]
population_mean = 7.5
t_statistic, p_value = stats.ttest_1samp(sample_data, population_mean)
Use Case: Determine if a sample of product reviews is representative of the entire customer population's satisfaction level.
Probability
Probability theory is like the building block of statistics. It's all about dealing with things we're not completely sure about and making guesses. In Python, the NumPy library has handy tools for working with probabilities.
Python Code Syntax:
from random import randint
probability_of_heads = sum(randint(0, 1) for _ in range(1000)) / 1000
Use Case: Calculate the probability of getting heads in a series of coin flips.
Sampling Techniques
Sampling is when we pick out a smaller group from a big bunch of data. We use different methods like random, stratified, or systematic sampling to make sure our small group is a good representation of the big group.
Python Code Syntax:
import random
sample = random.sample(data, 30)
Use Case: Randomly select a sample of customers to conduct a survey and make inferences about the entire customer base.
Regression Analysis
Regression analysis is about finding connections between different things. Like, using linear regression to guess one thing based on some other things. Python's Scikit-Learn library is great for doing this kind of analysis.
Python Code Syntax:
import statsmodels.api as sm
X = sm.add_constant(independent_variable)
model = sm.OLS(dependent_variable, X).fit()
Use Case: Predict the price of a house based on its size, location, and other features.
Hypothesis Testing
Hypothesis testing helps us make smart choices about data. We create two ideas, one called the "null" and the other "alternative," and then run tests to decide if the null idea should be kept or thrown out.
Python Code Syntax:
t_statistic, p_value = stats.ttest_ind(sample_group_A, sample_group_B)
Use Case: Determine if a new website design leads to a significant increase in user engagement.
Statistical Distributions
Statistical distributions describe the patterns and characteristics of data. Python libraries such as SciPy provide a wide range of distributions, including normal, binomial, and Poisson.
Python Code Syntax:
from scipy.stats import norm
data = [68, 70, 72, 74, 76]
mean = np.mean(data)
std_dev = np.std(data)
normal_dist = norm(loc=mean, scale=std_dev)
Use Case: Model the distribution of heights in a population to calculate percentiles and make predictions.
Time Series Analysis
Time series analysis is crucial for data with a temporal component. Libraries like Pandas and Statsmodels in Python are useful for time series analysis.
Python Code Syntax:
import pandas as pd
data = pd.read_csv('sales_data.csv', parse_dates=True, index_col='date')
Use Case: Analyze historical sales data to forecast future sales and identify seasonality trends.
To sum up, these statistical ideas are super important in data science. They help us find important stuff, make smart choices, and make predictions. Using Python, data scientists can use these ideas to learn cool things from data and make businesses better.
For those of you new to my newsletter - follow me on LinkedIn, hit me up on Twitter, and follow my Facebook Page.
To support me as I roll out more content weekly, click HERE to buy me a coffee.
This post is public so feel free to share it, Thank you for reading…